Lax-Wendroff Method#
The Differential Equation#
Condsider the one-dimensional hyperbolic Wave Equation:
with the initial conditions
and wrap around boundary conditions.
This notebook will implement the Lax-Wendroff method to appoximate the solution of the Wave Equation. The Lax-Wendroff method was designed by Peter Lax (https://en.wikipedia.org/wiki/Peter_Lax) and Burton Wendroff (https://en.wikipedia.org/wiki/Burton_Wendroff).
# LIBRARY
# vector manipulation
import numpy as np
# math functions
import math
# THIS IS FOR PLOTTING
%matplotlib inline
import matplotlib.pyplot as plt # side-stepping mpl backend
import warnings
warnings.filterwarnings("ignore")
Discete Grid#
The region \(\Omega\) is discretised into a uniform mesh \(\Omega_h\). In the space \(x\) direction into \(N\) steps giving a stepsize of
resulting in
and into \(N_t\) steps in the time \(t\) direction giving a stepsize of
resulting in
The Figure below shows the discrete grid points for \(N=10\) and \(Nt=100\), the known boundary conditions (green), initial conditions (blue) and the unknown values (red) of the Heat Equation.
N=20
Nt=10
h=2*np.pi/N
k=1/Nt
r=k/(h*h)
time_steps=10
time=np.arange(0,(time_steps+.5)*k,k)
x=np.arange(0,2*np.pi+h/2,h)
X, Y = np.meshgrid(x, time)
fig = plt.figure()
plt.plot(X,Y,'ro');
plt.plot(x,0*x,'bo',label='Initial Condition');
plt.xlim((-h,2*np.pi+h))
plt.ylim((-k,max(time)+k))
plt.xlabel('x')
plt.ylabel('time (ms)')
plt.legend(loc='center left', bbox_to_anchor=(1, 0.5))
plt.title(r'Discrete Grid $\Omega_h$ ',fontsize=24,y=1.08)
plt.show();
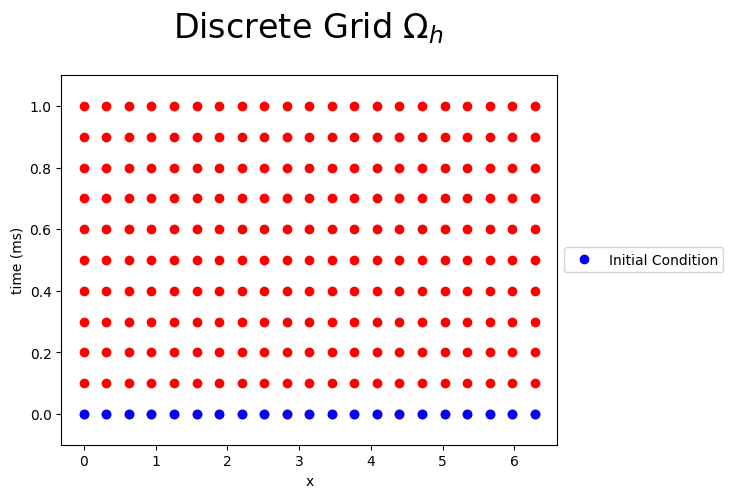
Initial Conditions#
The discrete initial conditions is,
The Figure below plots values of \(w[i,0]\) for the inital (blue) conditions for \(t[0]=0.\)
w=np.zeros((N+1,time_steps+1))
b=np.zeros(N-1)
# Initial Condition
for i in range (0,N+1):
w[i,0]=1-np.cos(x[i])
fig = plt.figure(figsize=(8,4))
plt.plot(x,w[:,0],'o:',label='Initial Condition')
plt.xlim([-0.1,max(x)+h])
plt.title('Intitial Condition',fontsize=24)
plt.xlabel('x')
plt.ylabel('w')
plt.legend(loc='best')
plt.show()
ipos = np.zeros(N+1)
ineg = np.zeros(N+1)
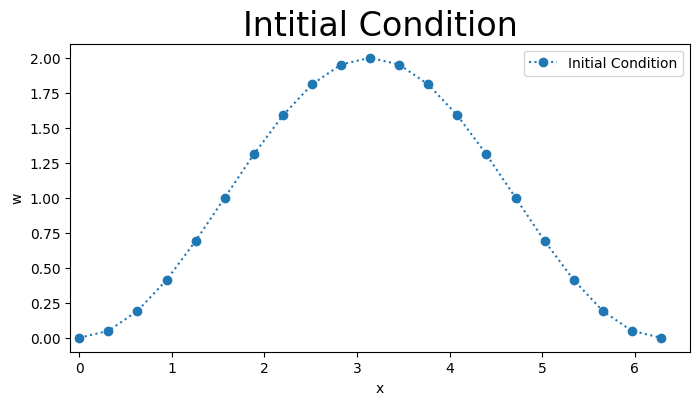
Boundary Conditions#
To account for the wrap-around boundary condtions
and
for i in range(0,N+1):
ipos[i] = int(i+1)
ineg[i] = int(i-1)
ipos[N] = 0
ineg[0] = N
Lax-Wendroff Method#
The Lax-Wendroff method for the Wave Equation is
Rearranging the equation we get
for \(i=0,...10\) where \(\lambda=\frac{k}{h}\).
This gives the formula for the unknown term \(w_{ij+1}\) at the \((ij+1)\) mesh points in terms of \(x[i]\) along the jth time row.
lamba=k/h
for j in range(0,time_steps):
for i in range (0,N+1):
w[i,j+1]=w[i,j]-lamba/2*(w[int(ipos[i]),j]-w[int(ineg[i]),j])+lamba*lamba/2*(w[int(ipos[i]),j]-2*w[i,j]+w[int(ineg[i]),j])/2
Results#
fig = plt.figure(figsize=(12,6))
plt.subplot(121)
for j in range (1,time_steps+1):
plt.plot(x,w[:,j],'o:')
plt.xlabel('x')
#plt.xticks(np.arange(len(x)), np.round(x,3),rotation=45)
plt.ylabel('w')
plt.subplot(122)
plt.imshow(w.transpose(), aspect='auto')
plt.xticks(np.arange(len(x)), np.round(x,3),rotation=45)
plt.yticks(np.arange(len(time)), np.round(time,2))
plt.xlabel('x')
plt.ylabel('time')
clb=plt.colorbar()
clb.set_label('Temperature (w)')
plt.suptitle('Numerical Solution of the Wave Equation'%(np.round(r,3)),fontsize=24,y=1.08)
fig.tight_layout()
plt.show()
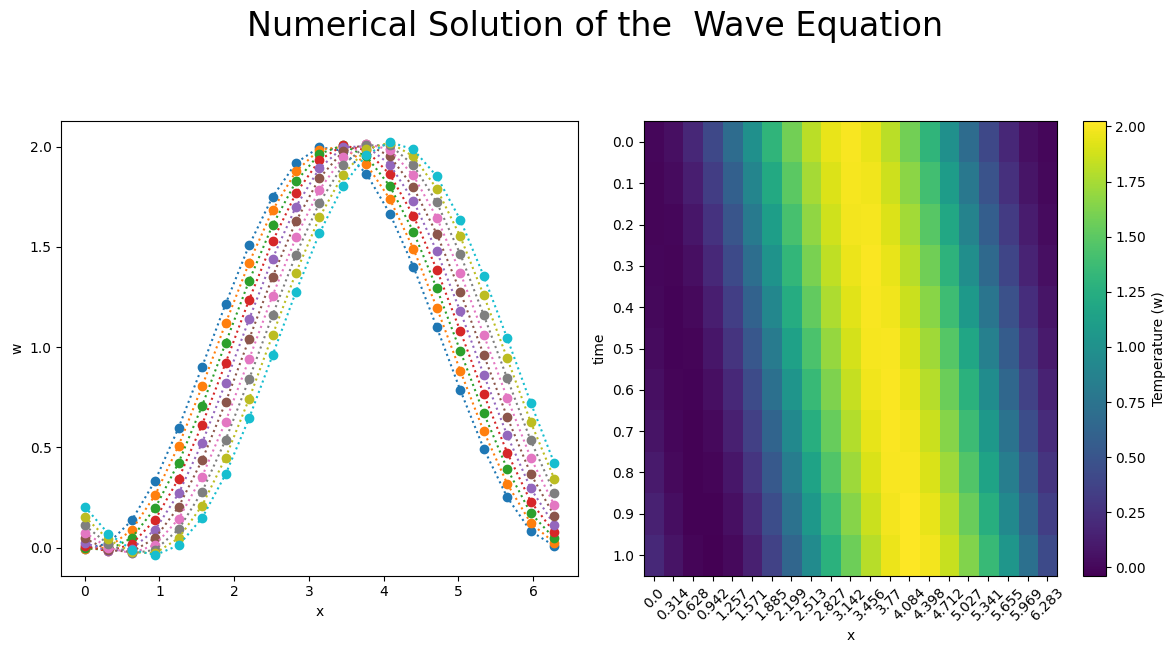