Question 6 Integrate and Fire#
from IPython.display import YouTubeVideo
YouTubeVideo('KDQpwb8MSOc', width=800, height=300)
YouTubeVideo('VSJvHiw6oo8', width=800, height=300)
Approximate the solution of the integrate and fire differential equation:
where \(E_L = -75\), \(\tau_m = 10\), \(R_m = 10\) and \(I(t)=0.01t\) and the initial condition \(V(-50) = -75\) using a stepsize of \(h=0.5\). Using the:
Euler Method;
2nd Order Taylor Method;
Heun’s Method (2nd Order Runge Kutta);
2-step Adams Bashforth Method.
## Library
import numpy as np
%matplotlib inline
import matplotlib.pyplot as plt # side-stepping mpl backend
import matplotlib.gridspec as gridspec # subplots
import warnings
warnings.filterwarnings("ignore")
Discrete Interval#
The continuous time \(a\leq t \leq b \) is discretised with stepsize \(h=0.5\) gives
Here the N is \(-50\leq t \leq 400\)
this gives the 901 discrete points:
This is generalised to
The plot below shows the discrete time steps.
### Setting up time
a=-50 # Start time point
b=400 # end time point
h=0.5 # step size
N=int((b-a)/(h)) # Number of gaps
t=np.arange(a,b+h/2,h) # -50, 400
fig = plt.figure(figsize=(10,4))
plt.plot(t,0*t,'.',color='red')
plt.xlim((a,b))
plt.title('Illustration of discrete time points for h=%s'%(h))
plt.plot();
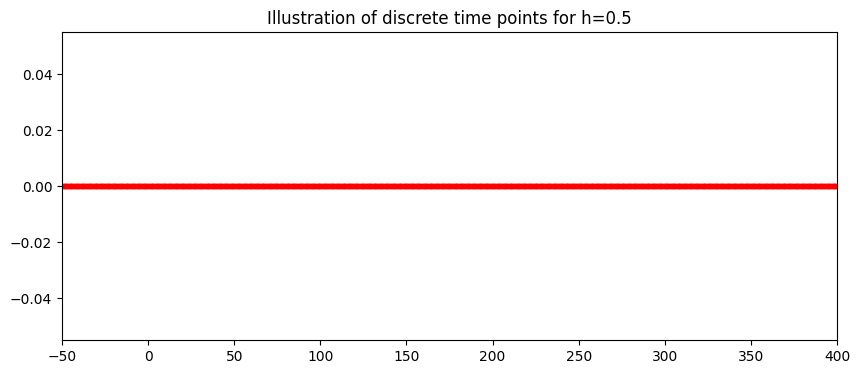
Euler Method#
The general form of the Euler method is
The Integrate and fire differential equation is transformed using the Euler method into a difference equation of the form
for \(i=0,1,...,899\) and where \(E_L = -75\), \(\tau_m = 10\), \(R_m = 10\) and \(I(t_i)=0.01t_i\) and the initial condition \(V(t_0=-50) = -75\) using a stepsize of \(h=0.5\). Putting in the values the difference equation is
In the solution, I will use Euler for Euler approximation, which gives:
Euler=np.zeros(N+1) # list of 901 0s
Euler[0]=-75 ## INITIAL CONDITION
for i in range (0,N): # 0,1,...899
Euler[i+1]=Euler[i]+h*(-(Euler[i]+75)+0.1*t[i])/10
fig = plt.figure(figsize=(12,5))
plt.plot(t,Euler,':',color='k')
plt.plot(t[0],Euler[0],'o',color='green')
plt.title('Approximate Euler Numerical Solution of the Integrate and Fire IVP h=%s'%(h))
plt.show()
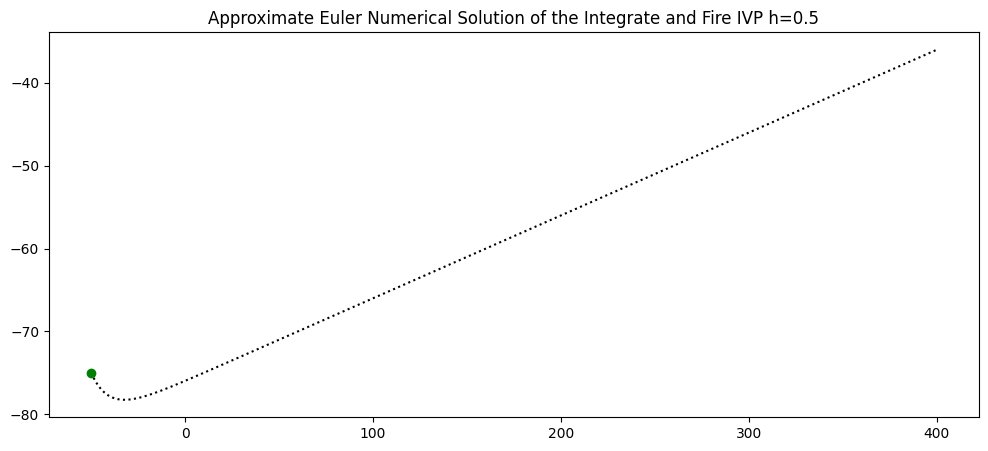
For simpilicty of numerical implimentation of the integrate and fire differential equation:
we re-arranged as:
where
# definint a function for f_IF that takes in t and V
def f_IF(t,V):
R_m=10
tau_m=10
E_L=-75
I=0.01*t
f=(-(V-E_L)+R_m*I)/tau_m
return f
2nd Order Taylor#
The general 2nd Order Taylor method for to the first order differential equation
numerical approximates \(y\) the at time point \(t_i\) as \(w_i\) with the formula:
where \(h\) is the stepsize. for \(i=0,...,N-1\).
For the Integrate and fire:
The 2nd Order Taylor will be of the form:
We need to get \(f_{IF}'\):
by definition of the IVP we have:
and \(I(t)=0.01t\)
In the solution, I will use Taylor for Taylor approximation, which gives:
which gives:
Taylor=np.zeros(N+1)
Taylor[0]=-75 ## INITIAL CONDITION
for i in range (0,N):
## ADD EQUATION DYNAMICS TO EQUATION
Taylor[i+1]=Taylor[i]+h*f_IF(t[i],Taylor[i])+h*h/2*(-f_IF(t[i],Taylor[i])/10+0.1/10)
Heun’s Method (2nd Order Runge Kutta)#
The general Heun’s method for to the first order differential equation
numerical approximates \(y\) the at time point \(t_i\) as \(w_i\) with the formula:
for \(i=0,...,N-1\), where
and
and \(h\) is the stepsize.
In the solution, I will use Huen for Heun approximation, which gives:
for \(i=0,...,N-1\), where
and
and \(h\) is the stepsize.
Heun=np.zeros(N+1)
Heun[0]=-75 ## INITIAL CONDITION
for i in range (0,N):
## ADD EQUATION DYNAMICS TO EQUATION
k1=f_IF(t[i],Heun[i])
k2=f_IF(t[i]+h,Heun[i]+h*k1)
Heun[i+1]=Heun[i]+h/2*(k1+k2)
2-step Adams-Bashforth#
The general 2-step Adams-Bashforth method for the first order differential equation
numerical approximates \(y\) the at time point \(t_i\) as \(w_i\) with the formula:
for \(i=1,...,N-1\), where
and \(h\) is the stepsize.
For the Integrate and Fire Equation we have
I will use AB to denote the approximate from the 2-step Adams-Bashforth:
for \(i=1,...,899\). We need to approximate \(AB[1]\) for the 2-step method. Here I will use the Heun solution at the second step i=1.
AB=np.zeros(N+1)
AB[0]=-75 ## INITIAL CONDITION
AB[1]=Heun[1] ## INITIAL HUEN CONDITION
for i in range (1,N):
AB[i+1]=AB[i]+h/2*(3*f_IF(t[i],AB[i])-f_IF(t[i-1],AB[i-1]))
fig = plt.figure(figsize=(12,5))
plt.plot(t,Euler,':',color='k',label='Euler')
plt.plot(t,Taylor,':',color='r',label='Taylor')
plt.plot(t,Heun,':',color='b',label='Heun')
plt.plot(t,AB,':',color='g',label='Adams-Bashforth')
plt.plot(t[0],Euler[0],'o',color='black',label='Initial Condition')
plt.legend(loc='best')
plt.xlabel('time (ms)')
plt.ylabel('Voltage')
plt.title('Numerical Solutions h=%s'%(h))
plt.show()
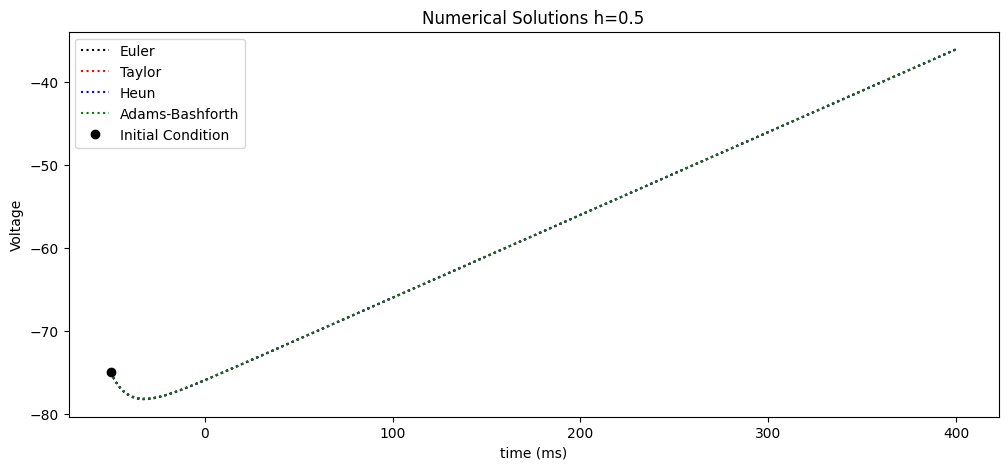
fig = plt.figure(figsize=(12,5))
plt.plot(t,Taylor-Euler,':',color='r',label='Taylor')
plt.plot(t,Heun-Euler,':',color='b',label='Heun')
plt.plot(t,AB-Euler,':',color='g',label='Adams-Bashforth')
plt.legend(loc='best')
plt.title('Numerical Solutions versus Euler h=%s'%(h))
plt.show()
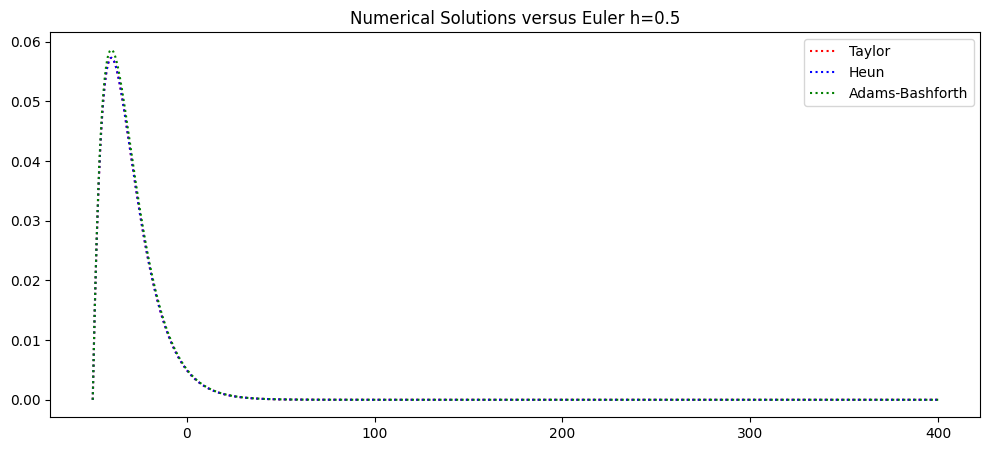